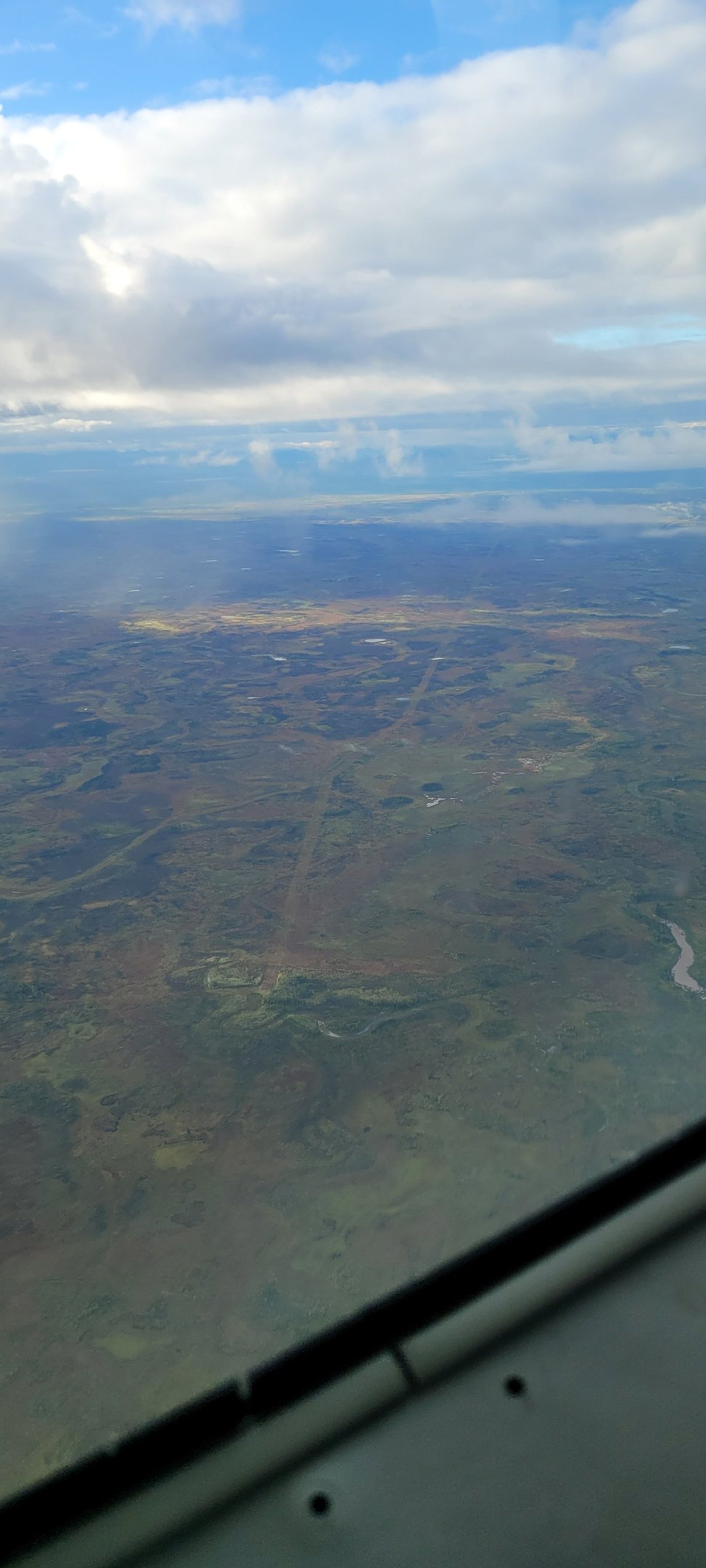
Tim Illguth's
Portfolio Website
Work Experience
Explore Tim Illguth's extensive work experience, showcasing his expertise in blockchain, Linux, networking, and DevOps through various projects and collaborations with esteemed professionals and over a decades work with NASA
Portfolio
Professional Background Overview
Tim's portfolio highlights his certifications and impactful projects in technology and education.
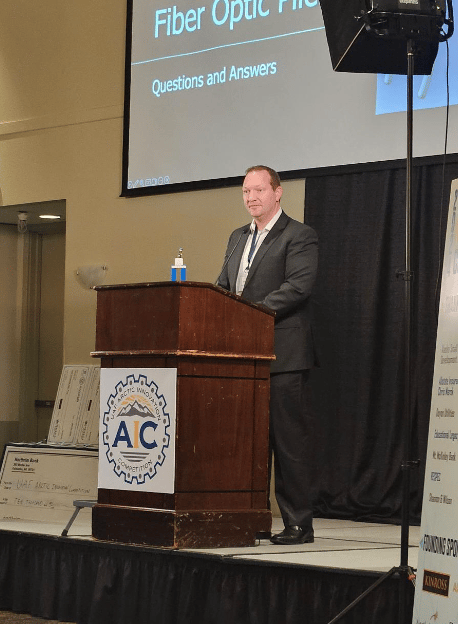
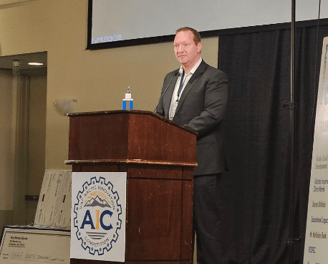
Certifications and Skills
Experience. With our intuitive design and user-friendly interface, your website will captivate visitors.
Get in Touch
Please feel free to reach out to me with this form, message me on LinkedIn or email me!
Contact
timillguth@outlook.com